Designing the Sign-In Page for the Flutter Ecommerce App
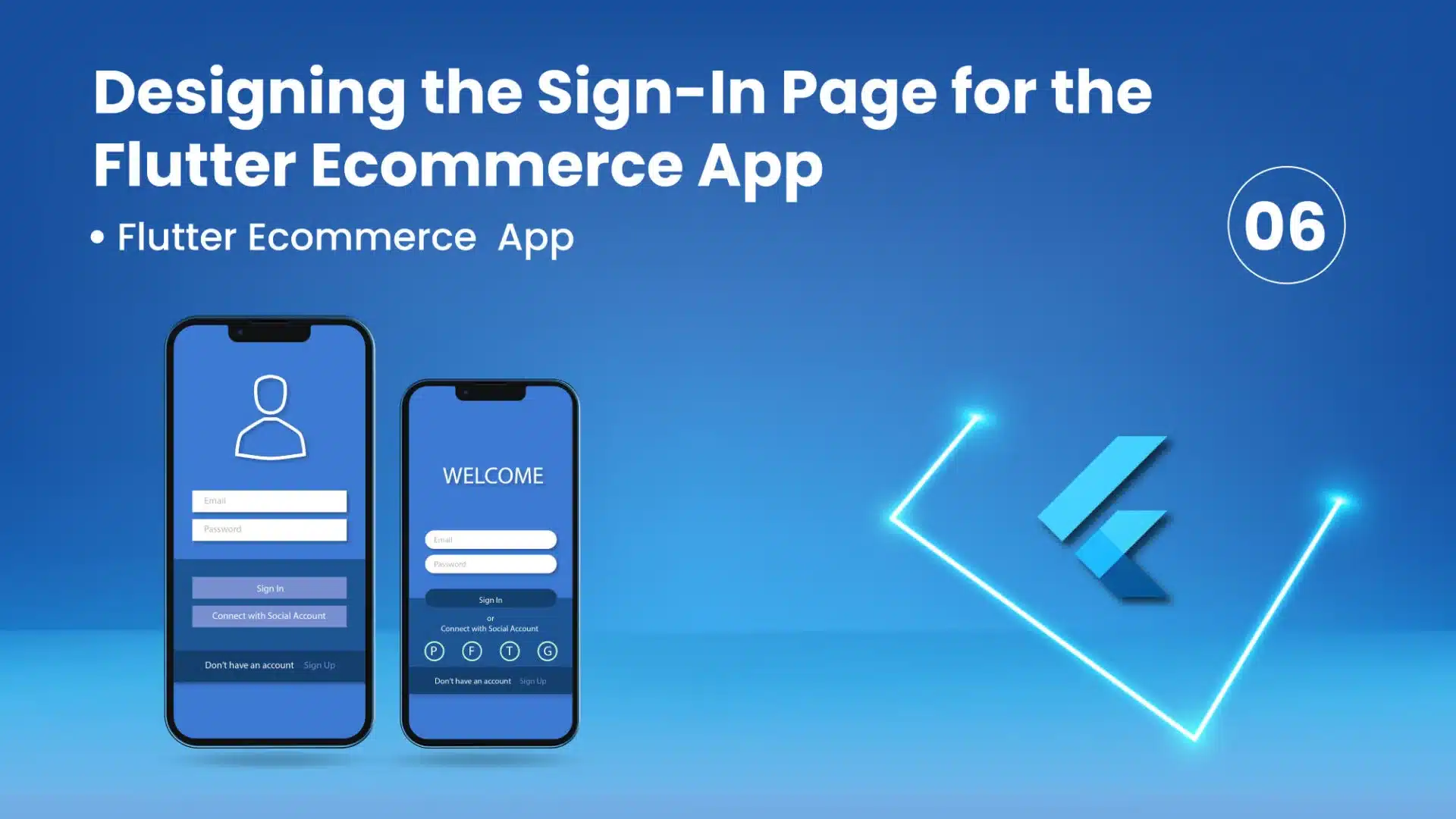
25 July
In this section, we will focus on designing the sign-in page for our Flutter ecommerce app. The sign-in page is an essential component of the authentication process, allowing users to log in to their accounts. We will create a visually appealing and user-friendly sign-in page that includes input fields for email and password, a sign-in button, and optional links for password recovery or creating a new account. By following the principles of responsive design, we will ensure that the page adapts seamlessly to different screen sizes. Let’s begin designing the sign-in page to provide a smooth and secure login experience for our app users.
import 'package:clickmart/view/authentication/forgot_pass.dart'; import 'package:clickmart/view/authentication/sign_up.dart'; import 'package:flutter/material.dart'; import 'package:flutter_signin_button/flutter_signin_button.dart'; class SignIn extends StatefulWidget { const SignIn({super.key}); @override State<SignIn> createState() => _SignInState(); } class _SignInState extends State<SignIn> { // Form Key final GlobalKey<FormState> _formKey = GlobalKey<FormState>(); // TextForm Controller TextEditingController emailController = TextEditingController(); TextEditingController passwordController = TextEditingController(); // Form Validation bool _validateAndSave() { final form = _formKey.currentState; if (form!.validate()) { form.save(); return true; } return false; } void _validateAndSubmit() { if (_validateAndSave()) { // TODO: Perform sign-in logic here } } @override Widget build(BuildContext context) { return WillPopScope( onWillPop: () { return Future.value(false); }, child: Scaffold( appBar: AppBar( automaticallyImplyLeading: false, title: const Text('Sign In'), ), body: Container( padding: const EdgeInsets.all(16.0), child: Form( key: _formKey, child: Column( crossAxisAlignment: CrossAxisAlignment.stretch, children: <Widget>[ TextFormField( controller: emailController, decoration: const InputDecoration(labelText: 'Email'), validator: (value) { if (value!.isEmpty) { return 'Please enter your email'; } return null; }, onSaved: (value) => emailController.text = value!, ), const SizedBox(height: 16.0), TextFormField( controller: passwordController, decoration: const InputDecoration(labelText: 'Password'), obscureText: true, validator: (value) { if (value!.isEmpty) { return 'Please enter your password'; } return null; }, onSaved: (value) => passwordController.text = value!, ), const SizedBox(height: 16.0), ElevatedButton( onPressed: _validateAndSubmit, child: const Text('Sign In'), ), const SizedBox(height: 16.0), Center( child: GestureDetector( onTap: _navigateToForgotPass, child: const Text( 'Forgot Password ?', style: TextStyle( fontWeight: FontWeight.bold, color: Colors.blue, ), ), ), ), const SizedBox(height: 16.0), Center( child: GestureDetector( onTap: _navigateToSignUp, child: RichText( text: const TextSpan( text: 'Don\'t have an account? ', style: TextStyle(color: Colors.black), children: <TextSpan>[ TextSpan( text: 'Sign Up', style: TextStyle( fontWeight: FontWeight.bold, color: Colors.blue, ), ), ], ), ), ), ), const SizedBox(height: 16.0), const Center( child: Text('Or'), ), const SizedBox(height: 16.0), SignInButton( Buttons.Google, onPressed: () { // TODO: Implement Google sign-in logic here }, ), ], ), ), ), ), ); } // Goto SignUp Page void _navigateToSignUp() { Navigator.pushReplacement( context, MaterialPageRoute(builder: (context) => const SignUp()), ); } // Goto Forgot Pass Page void _navigateToForgotPass() { Navigator.pushReplacement( context, MaterialPageRoute(builder: (context) => const ForgotPassword()), ); } }
OUTPUT :
Conclusion
Designing a well-crafted sign-in page is a crucial step in creating a seamless and secure authentication experience for users in our Flutter ecommerce app. Throughout this section, we have focused on the following key elements to achieve an engaging and user-friendly sign-in page:
- Visual Appeal: By employing thoughtful design choices, we have ensured that the sign-in page is visually appealing, creating a positive first impression for users.
- User-Friendly Input Fields: The inclusion of input fields for email and password allows users to easily provide their credentials for login.
- Intuitive Navigation: Optional links for password recovery and creating a new account offer users convenient access to essential account management features.
- Responsive Design: By adhering to responsive design principles, our sign-in page seamlessly adapts to various screen sizes, providing a consistent experience for all users.
- Security: As security is paramount in authentication, we will further enhance the page with Firebase Authentication to ensure robust and secure user login.
The sign-in page sets the tone for the overall user experience and plays a crucial role in establishing user trust and engagement. By creating an intuitive and visually appealing sign-in page, we are ready to deliver a smooth and secure login process to our app users.
Next, we will explore designing other essential pages, such as the sign-up page, product details page, user profile, and notification page, to provide a comprehensive and captivating ecommerce app experience. By continuing to apply best design practices and thoughtful user interface considerations, we will create an ecommerce app that stands out and leaves a lasting impression on our users. Let’s continue our journey to design an ecommerce app that excels in both aesthetics and functionality!