How to Build a High-Performance App Using React Native
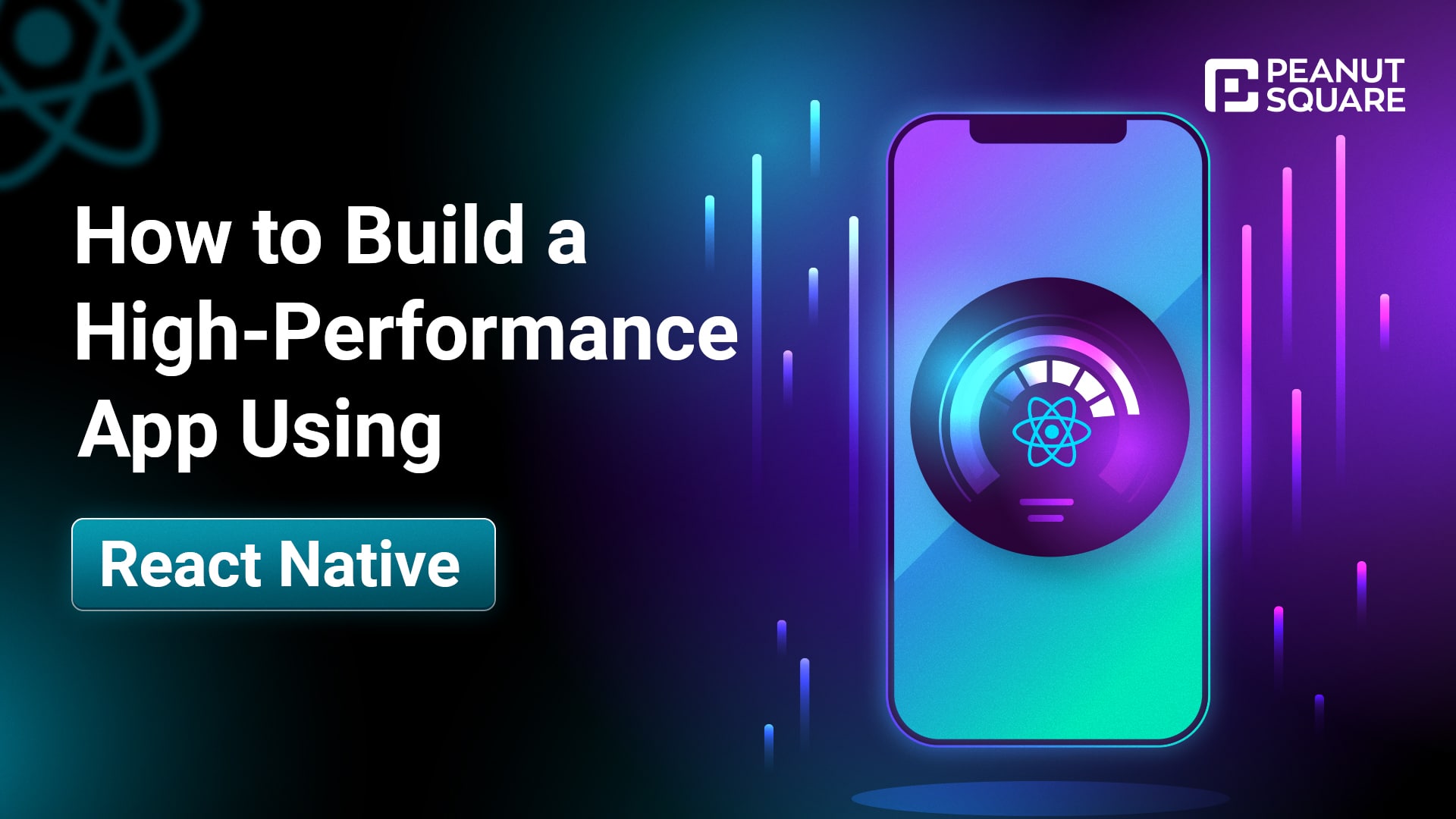
19 January
React Native has become one of the most popular frameworks for building cross-platform mobile applications. Its ability to write code once and deploy it on both iOS and Android makes it an attractive option for developers.
However, to ensure your app performs smoothly and efficiently, it’s essential to follow best practices. In this blog, we’ll explore key strategies to build high-performance React Native apps.
1. Optimize Your JavaScript Code
React Native apps heavily rely on JavaScript. Writing clean and efficient JavaScript code is crucial for performance. Here are some tips:
- Avoid Inline Functions: Define functions outside of the render method to prevent unnecessary re-renders.
- Use PureComponent or Memo: These can prevent re-renders for components that don’t depend on updated props or state.
- Minimize State Updates: Use batching to minimize the number of updates to the state and re-renders.
2. Leverage Native Modules and Components
While React Native offers a lot of pre-built components, you might encounter scenarios where a native module is more efficient:
- Use Native Modules for Heavy Tasks: For complex calculations or animations, consider implementing native modules.
- Optimize List Rendering: Use FlatList or SectionList for rendering large data sets instead of ScrollView.
3. Optimize Image Rendering
Images can significantly impact app performance if not handled properly. Consider the following:
- Use Correct Image Sizes: Resize images to the exact dimensions required before adding them to the project.
- Leverage Lazy Loading: Load images only when they come into view using libraries like
react-native-fast-image
. - Cache Images: Use caching strategies to avoid repeatedly downloading the same images.
4. Efficient Navigation
Navigation plays a critical role in app performance. Choose the right library and configuration:
- React Navigation: Use libraries like React Navigation with Stack Navigator, but enable screens to be detached or removed from memory when not in use.
- React Native Screens: Leverage this library to enhance the performance of stack-based navigation.
5. Reduce Overhead with Native Drivers for Animations
Animations can consume significant resources. Use the following techniques to improve their performance:
- Use Native Driver: Use the
useNativeDriver
property for animations to offload processing to the native thread. - Avoid Complex Animations: Keep animations simple and lightweight to avoid high memory usage.
6. Minimize Network Requests
Optimizing API calls and data fetching improves app performance:
- Batch API Calls: Combine multiple API requests into a single call wherever possible.
- Use Pagination: Implement pagination or infinite scrolling for large data sets.
- Cache API Responses: Use libraries like Redux Persist or AsyncStorage to store frequently used data.
7. Monitor App Performance
Continuous monitoring helps you identify and address performance bottlenecks:
- Use Profiling Tools: Leverage tools like React Native Performance Monitor, Flipper, or Android Studio Profiler.
- Track Memory Usage: Identify memory leaks and fix them promptly.
- Analyze Render Times: Use
why-did-you-render
to find unnecessary re-renders.
8. Optimize Third-Party Dependencies
Using too many third-party libraries can slow down your app. To avoid this:
- Audit Dependencies: Regularly review the libraries in your project and remove unused ones.
- Choose Lightweight Libraries: Opt for libraries with minimal overhead.
- Avoid Overlapping Libraries: Use a single library for similar tasks (e.g., date formatting).
9. Use Hermes for Android
Hermes is a JavaScript engine optimized for React Native apps:
- Enable Hermes: Add Hermes to your project by configuring your
android/app/build.gradle
file. - Faster Load Times: Hermes reduces app start-up time and improves overall performance.
10. Build for Release
Always test and build your app for production to achieve optimal performance:
- Minify JavaScript: Enable minification and obfuscation in your build process.
- Use Release Builds: Test the app in release mode to evaluate its true performance.
Conclusion
Building a high-performance React Native app requires attention to detail and adherence to best practices. By optimizing your code, leveraging native modules, and continuously monitoring performance, you can ensure your app delivers a smooth and seamless user experience.
With the right strategies in place, React Native can help you create apps that rival native performance.