Your Complete Guide to React Native Navigation in 2025
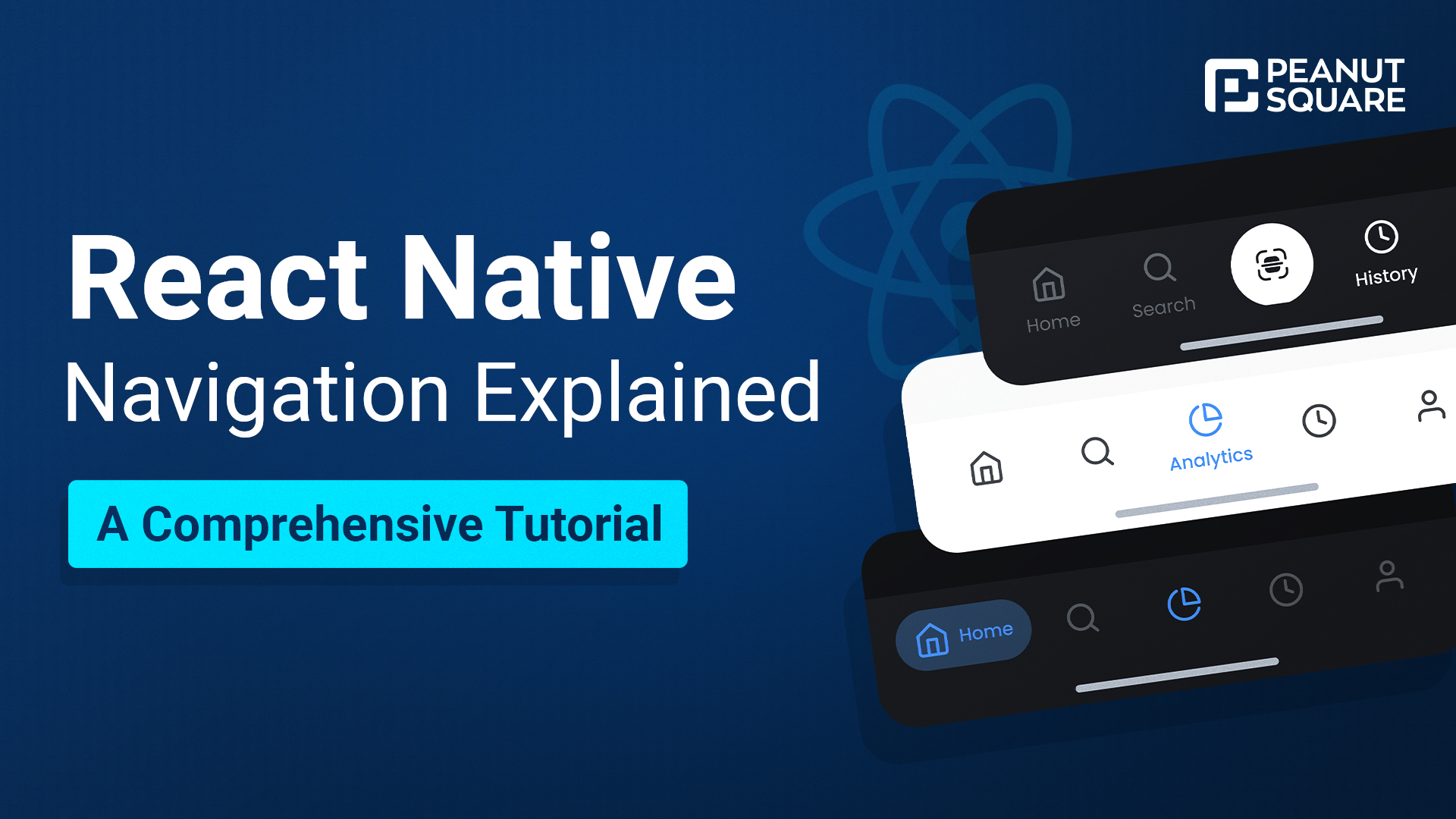
4 January
Navigation is a core aspect of mobile app development, and React Native offers various libraries to implement smooth and efficient navigation. Whether you’re building a simple app or a complex one with nested navigators, this tutorial will guide you through the basics and advanced concepts of React Native navigation.
1. Why Navigation is Important in React Native Apps
Navigation helps users move between different screens or views in your app. A well-implemented navigation system ensures:
- Intuitive User Flow: Makes the app easier to use.
- Efficient Resource Management: Loads only the necessary screens to optimize performance.
- Consistency: Provides a seamless user experience across iOS and Android platforms.
React Native’s navigation ecosystem is diverse, but some of the most popular libraries are:
- React Navigation
- React Native Navigation (by Wix)
- React Router Native
2. Setting Up React Navigation
React Navigation is one of the most widely used libraries for navigation in React Native. It offers a wide range of navigators, including stack, tab, and drawer navigators.
Installation
To get started with React Navigation, install the required packages:
npm install @react-navigation/native react-native-screens react-native-safe-area-context react-native-gesture-handler react-native-reanimated react-native-vector-icons
npm install @react-navigation/stack
Wrap your app in the NavigationContainer:
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
export default function App() {
return (
<NavigationContainer>
{/* Navigator goes here */}
</NavigationContainer>
);
}
3. Implementing Basic Navigation
Stack Navigator
A stack navigator allows you to navigate between screens in a stack-like fashion (think of it as a deck of cards).
import { createStackNavigator } from '@react-navigation/stack';
import HomeScreen from './HomeScreen';
import DetailsScreen from './DetailsScreen';
const Stack = createStackNavigator();
function MyStack() {
return (
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
);
}
export default function App() {
return (
<NavigationContainer>
<MyStack />
</NavigationContainer>
);
}
Tab Navigator
Tab navigation is useful for apps with multiple sections, such as social media apps.
npm install @react-navigation/bottom-tabs
Example:
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
import HomeScreen from './HomeScreen';
import ProfileScreen from './ProfileScreen';
const Tab = createBottomTabNavigator();
function MyTabs() {
return (
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeScreen} />
<Tab.Screen name="Profile" component={ProfileScreen} />
</Tab.Navigator>
);
}
Drawer Navigator
Drawer navigation adds a sidebar menu for easy navigation.
npm install @react-navigation/drawer
Example:
import { createDrawerNavigator } from '@react-navigation/drawer';
import HomeScreen from './HomeScreen';
import SettingsScreen from './SettingsScreen';
const Drawer = createDrawerNavigator();
function MyDrawer() {
return (
<Drawer.Navigator>
<Drawer.Screen name="Home" component={HomeScreen} />
<Drawer.Screen name="Settings" component={SettingsScreen} />
</Drawer.Navigator>
);
}
4. Handling Navigation Props
React Navigation passes navigation
and route
props to each screen component:
navigation
: Contains methods likenavigate
,goBack
, andreset
.route
: Provides access to parameters passed to the screen.
Example of navigating with parameters:
navigation.navigate('Details', { itemId: 42 });
Accessing parameters:
const { itemId } = route.params;
5. Nested Navigators
You can combine different navigators to create a complex navigation structure:
function App() {
return (
<NavigationContainer>
<Drawer.Navigator>
<Drawer.Screen name="Main" component={MainTabs} />
</Drawer.Navigator>
</NavigationContainer>
);
}
function MainTabs() {
return (
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeStack} />
</Tab.Navigator>
);
}
function HomeStack() {
return (
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
</Stack.Navigator>
);
}
6. Optimizing Performance
To ensure smooth navigation:
- Enable Native Screens: Install and configure
react-native-screens
for better memory management. - Use Lazy Loading: Load screens only when they are accessed by setting
lazy
totrue
. - Detach Screens: For stack navigators, use
detachInactiveScreens
to reduce memory usage.
7. Debugging Navigation Issues
If you encounter issues with navigation:
- Use the React Navigation DevTools to inspect the navigation stack.
- Check for proper installation of dependencies.
- Wrap the app in
GestureHandlerRootView
if you’re facing gesture-related issues.
How Peanut Square Helps You Build Your Dream App
Building a smooth and intuitive navigation system can be complex, but you don’t have to do it alone! At Peanut Square LLP, we specialize in building high-performance mobile apps tailored to your unique business needs. Whether you’re looking to integrate advanced navigation features, optimize your app’s performance, or bring your vision to life, our team of skilled developers is here to help.
Contact Peanut Square LLP today to transform your ideas into reality and create a seamless experience for your users. Let’s build something amazing together!
Conclusion
React Native navigation is a powerful tool that enables developers to create seamless and intuitive user experiences. By mastering different navigators, handling nested structures, and optimizing performance, you can build robust navigation systems for your apps.
With this comprehensive guide and support from Peanut Square LLP, you’re ready to implement efficient navigation in your next React Native project.